Originally published November 14, 2016 @ 11:10 pm
Below is a quick script that will replace specified strings in binary files with random values. A word of caution: the script will preserve the file’s original ctime
by briefly changing system time. Obviously, this requires root
access and may cause issues with some applications. If this is not a feature you need, you can just comment out the time_set
function on line 58.
Download and install the script:
d="/var/adm/bin" n="patch_binary" mkdir -p ${d} cd ${d} wget -O ${d}/${n}.zip http://www.krazyworks.com/wp-content/uploads/2016/11/${n}.zip unzip ${d}/${n}.zip chmod 755 ${d}/${n}.sh ln -s ${d}/${n}.sh /usr/bin/patchbin
Example:
This will (hopefully) replace strings jdoe1
and 10.10.5.13
in the two binary log files
patchbin -k "jdoe1 10.10.5.13" -f "/var/log/wtmp /var/log/lastlog"
The script:
#!/bin/bash while getopts ":k:f:" opt do case ${opt} in k) set -f IFS=' ' array_k=(${OPTARG}) ;; f) set -f IFS=' ' array_f=(${OPTARG}) ;; *) exit 1 ;; esac done if [ "${#array_k[@]}" -eq 0 ] || [ "${#array_f[@]}" -eq 0 ] then exit 1 fi time_set() { curdate=$(date) && date -s "${ctime}" >/dev/null 2>&1 && touch "${i}" && date -s "${curdate}" >/dev/null 2>&1 } r="${RANDOM}" for i in "${array_f[@]}" do echo "${i}" if [ -f "${i}" ] then ctime=$(stat -c %z "${i}") for u in "${array_k[@]}" do strings ${i} | grep "${u}" | sort -u -r | while read os do ns="$(sed "s/${u}/$(tr -dc 'a-zA-Z0-9' </dev/urandom | fold -w $(echo ${#u}) | head -n 1)/g" <<<"${os}")" osh="$(echo -n ${os} | xxd -g 0 -u -ps -c 256 | tr -d '\n')00" nsh="$(echo -n ${ns} | xxd -g 0 -u -ps -c 256 | tr -d '\n')00" hexdump -ve '1/1 "%.2X"' "${i}" | sed -r "s/${osh}/${nsh}/g" | xxd -r -p > "${i}_${r}" /bin/mv -f "${i}_${r}" "${i}" done done time_set fi done
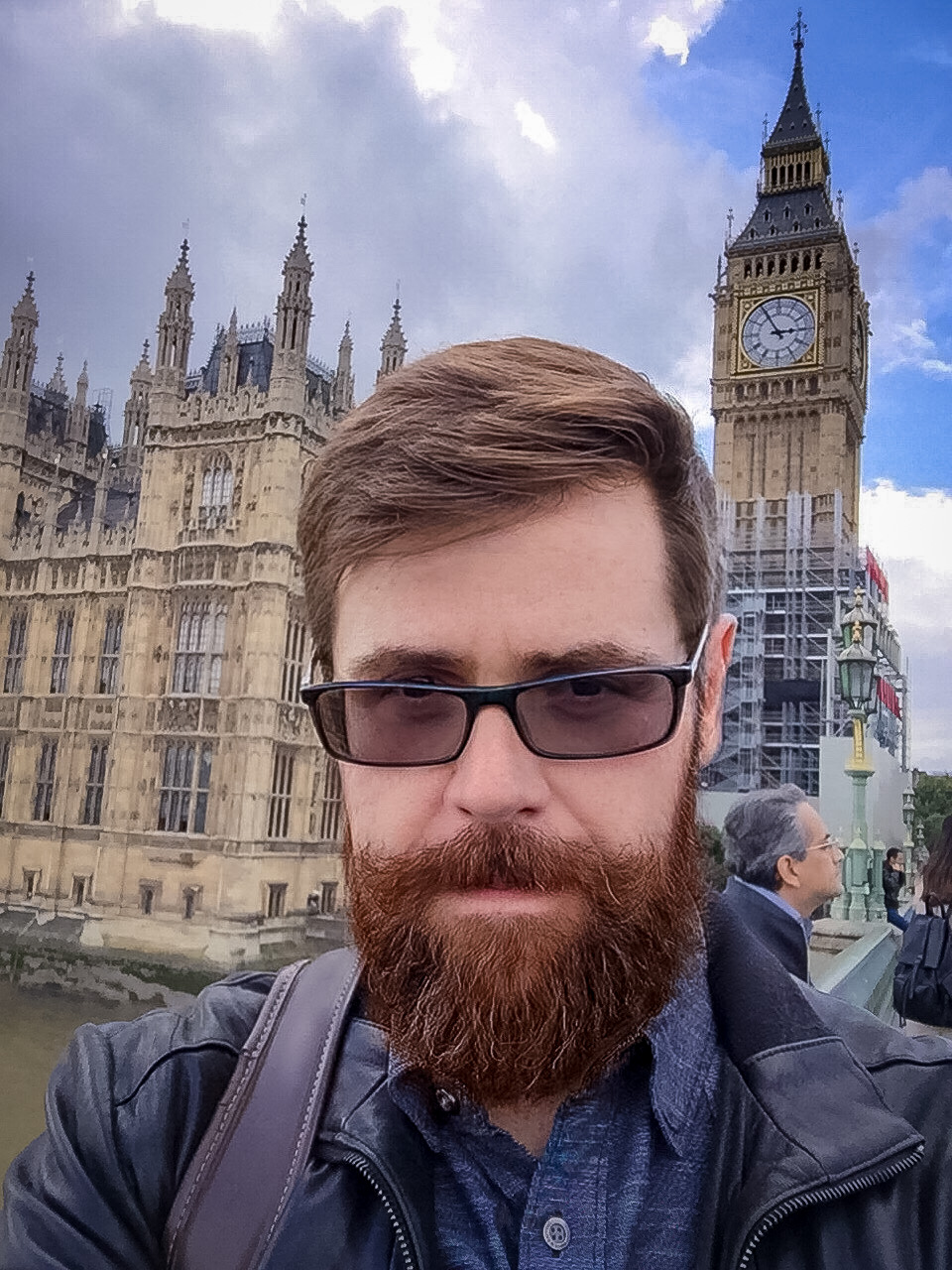
Experienced Unix/Linux System Administrator with 20-year background in Systems Analysis, Problem Resolution and Engineering Application Support in a large distributed Unix and Windows server environment. Strong problem determination skills. Good knowledge of networking, remote diagnostic techniques, firewalls and network security. Extensive experience with engineering application and database servers, high-availability systems, high-performance computing clusters, and process automation.